4. Document
This section provides four examples of how to define new and call existing properties, geometries, selection sets, and conditions in STKO.
4.1. Properties
In STKO, properties are accessible via the Python API. To start, import the PyMpc module and load the cae document as a variable in Python:
from PyMpc import *
doc = App.caeDocument()
You can then query the doc
variable for its properties.
For example, to view all the physical properties defined in the document:
from PyMpc import *
App.clearTerminal()
doc = App.caeDocument()
for item in dir(doc):
print(item)
This will output a list of all the physical properties, along with their attributes and methods.
__class__, __delattr__, __dict__, __dir__, __doc__, __eq__, __format__, __ge__, __getattribute__, __gt__, __hash__, __init__, __init_subclass__, __instance_size__, __le__, __lt__, __module__, __ne__, __new__, __reduce__, __reduce_ex__, __repr__, __setattr__, __sizeof__, __str__, __subclasshook__, __weakref__, addAnalysisStep, addCondition, addCustomDrawableEntity, addDefinition, addElementProperty, addGeometry, addInteraction, addLocalAxes, addPhysicalProperty, addSelectionSet, analysisSteps, clearCustomDrawableEntities, commitChanges, conditions, definitions, dirty, documentName, elementProperties, fileName, geometries, getAnalysisStep, getCondition, getDefinition, getElementProperty, getFileExtension, getFileFilter, getGeometry, getInteraction, getLocalAxes, getPhysicalProperty, getSelectionSet, interactions, localAxes, mesh, meshControls, metaDataAnalysisStep, metaDataCondition, metaDataDefinition, metaDataElementProperty, metaDataPhysicalProperty, new, physicalProperties, randomizeMaterialColors, registerMetaDataAnalysisStep, registerMetaDataCondition, registerMetaDataDefinition, registerMetaDataElementProperty, registerMetaDataPhysicalProperty, registeredMetaDataAnalysisSteps, registeredMetaDataConditions, registeredMetaDataDefinitions, registeredMetaDataElementProperties, registeredMetaDataPhysicalProperties, removeCustomDrawableEntity, scene, selectionSets, solverName, solverSettings, unregisterMetaDataAll, unregisterMetaDataAnalysisStep, unregisterMetaDataAnalysisSteps, unregisterMetaDataCondition, unregisterMetaDataConditions, unregisterMetaDataDefinition, unregisterMetaDataDefinitions, unregisterMetaDataElementProperties, unregisterMetaDataElementProperty, unregisterMetaDataPhysicalProperties, unregisterMetaDataPhysicalProperty, uuid
These are all the different callable members of a Cae Document.
For example the physicalProperties
attribute is a list of all the physical properties defined in the document.
You can view it like this:
from PyMpc import *
App.clearTerminal()
doc = App.caeDocument()
for k,v in doc.physicalProperties.items():
print(k,v)
print(dir(v.XObject))
If you are in an empty file, the output will be blank, but if you have physical properties defined in the document, you will get something like this:
1 <PyMpc.MpcProperty object at 0x0000029C1262FDF0>
['Xgroup', 'Xnamespace', '__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__', 'attributes', 'completeName', 'createInstanceOf', 'getAttribute', 'name', 'parent']
4.2. Geometries
In this example, we will create new geometries in the Preprocessor using the PyMpc API.
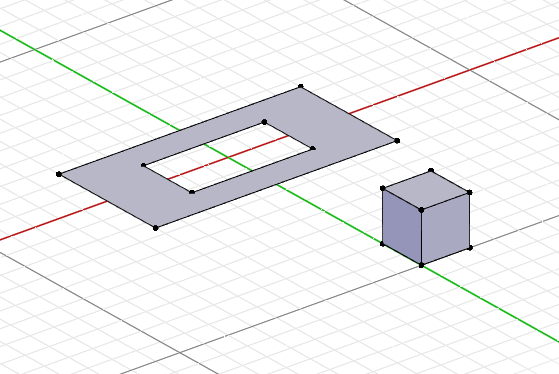
Fig. 4.2.1 Geometry created with the following script
First, import the necessary modules and load the cae document:
from PyMpc import *
from random import random
App.clearTerminal()
doc = App.caeDocument()
Next, initialize the id at 0 and set an if loop to check if there are existing geometries and reset the id to the greatest existing index plus one:
id = 0
if len(doc.geometries) > 0:
id = doc.geometries.keys()[-1]
print(id)
def get_id():
global id
id += 1
return id
Then, define a function to add geometries to the document, commit the changes, and define the document as dirty:
def add_geom(geom):
doc.addGeometry(geom)
doc.commitChanges()
doc.dirty = True
App.runCommand("Regenerate")
App.processEvents()
Create a new function to draw a wire with an argument scale:
def wire(scale):
#Define the 4 vertices of the outer wire (each vertex coordinates in expressed as a function of scale):
v1 = FxOccBuilder.makeVertex(-50*scale, -25*scale, 0)
add_geom(MpcGeometry(get_id(), "V1", v1))
v2 = FxOccBuilder.makeVertex(50*scale, -25*scale, 0)
add_geom(MpcGeometry(get_id(), "V2", v2))
v3 = FxOccBuilder.makeVertex(50*scale, 25*scale, 0)
add_geom(MpcGeometry(get_id(), "V3", v3))
v4 = FxOccBuilder.makeVertex(-50*scale, 25*scale, 0)
add_geom(MpcGeometry(get_id(), "V4", v4))
#Define the edges connecting the vertices:
e1 = FxOccBuilder.makeEdge(v1, v2)
add_geom(MpcGeometry(get_id(), "E1", e1))
e2 = FxOccBuilder.makeEdge(v2, v3)
add_geom(MpcGeometry(get_id(), "E2", e2))
e3 = FxOccBuilder.makeEdge(v3, v4)
add_geom(MpcGeometry(get_id(), "E3", e3))
e4 = FxOccBuilder.makeEdge(v4, v1)
add_geom(MpcGeometry(get_id(), "E4", e4))
#Create the wire with the makeWire function from the sub-module FxOccBuilder:
w1 = FxOccBuilder.makeWire([e1, e2, e3, e4])
add_geom(MpcGeometry(get_id(), "W1", w1))
return w1
Create the two wires and a face with a hole:
w1 = wire(1.0)
w2 = wire(0.5)
f1 = FxOccBuilder.makeFace(w1, [w2])
add_geom(MpcGeometry(get_id(), "F1", f1))
Similarly, create a solid by defining its vertices, edges, wires, faces, and shells:
v1 = FxOccBuilder.makeVertex(0 , -100, 0) #vertices
v2 = FxOccBuilder.makeVertex(20, -100, 0)
v3 = FxOccBuilder.makeVertex(20, -80, 0)
v4 = FxOccBuilder.makeVertex(0 , -80, 0)
v5 = FxOccBuilder.makeVertex(0 , -100, 20)
v6 = FxOccBuilder.makeVertex(20, -100, 20)
v7 = FxOccBuilder.makeVertex(20, -80, 20)
v8 = FxOccBuilder.makeVertex(0 , -80, 20)
e1 = FxOccBuilder.makeEdge(v1,v2) #bottom edges, wire, face
e2 = FxOccBuilder.makeEdge(v2,v3)
e3 = FxOccBuilder.makeEdge(v3,v4)
e4 = FxOccBuilder.makeEdge(v4,v1)
w1 = FxOccBuilder.makeWire([e1,e2,e3,e4])
f1 = FxOccBuilder.makeFace(w1)
e5 = FxOccBuilder.makeEdge(v5,v6) #top edges, wire, face
e6 = FxOccBuilder.makeEdge(v6,v7)
e7 = FxOccBuilder.makeEdge(v7,v8)
e8 = FxOccBuilder.makeEdge(v8,v5)
w2 = FxOccBuilder.makeWire([e5,e6,e7,e8])
f2 = FxOccBuilder.makeFace(w2)
e9 = FxOccBuilder.makeEdge(v1,v5) #front edges, wire, face
e10 = FxOccBuilder.makeEdge(v2,v6)
w3 = FxOccBuilder.makeWire([e1,e10,e5,e9])
f3 = FxOccBuilder.makeFace(w3)
e11 = FxOccBuilder.makeEdge(v4,v8) #back edges, wire, face
e12 = FxOccBuilder.makeEdge(v3,v7)
w4 = FxOccBuilder.makeWire([e3,e12,e7,e11])
f4 = FxOccBuilder.makeFace(w4)
w5 = FxOccBuilder.makeWire([e4,e9,e8,e11]) #left wire, face
f5 = FxOccBuilder.makeFace(w5)
w6 = FxOccBuilder.makeWire([e2,e10,e6,e12]) #right wire, face
f6 = FxOccBuilder.makeFace(w6)
s1 = FxOccBuilder.makeShell([f1,f2,f3,f4,f5,f6]) #shell
so1 = FxOccBuilder.makeSolid(s1) #solid
add_geom(MpcGeometry(get_id(), "S1", so1))
4.3. Selection Sets
Note
Coming soon.
4.4. Conditions
Note
Coming soon.